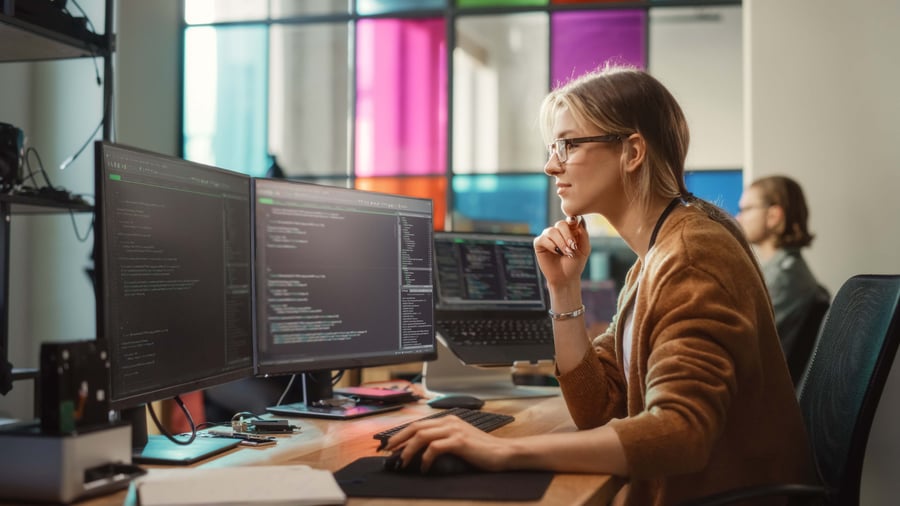
Customer portal
My Pages
The My Pages Portal is designed with our customers in mind, aiming to provide a seamless and exceptional experience. Our portal is a centralized hub where you can access various features and functions. You can among other things find:
- technical support
- technical articles
- customer support