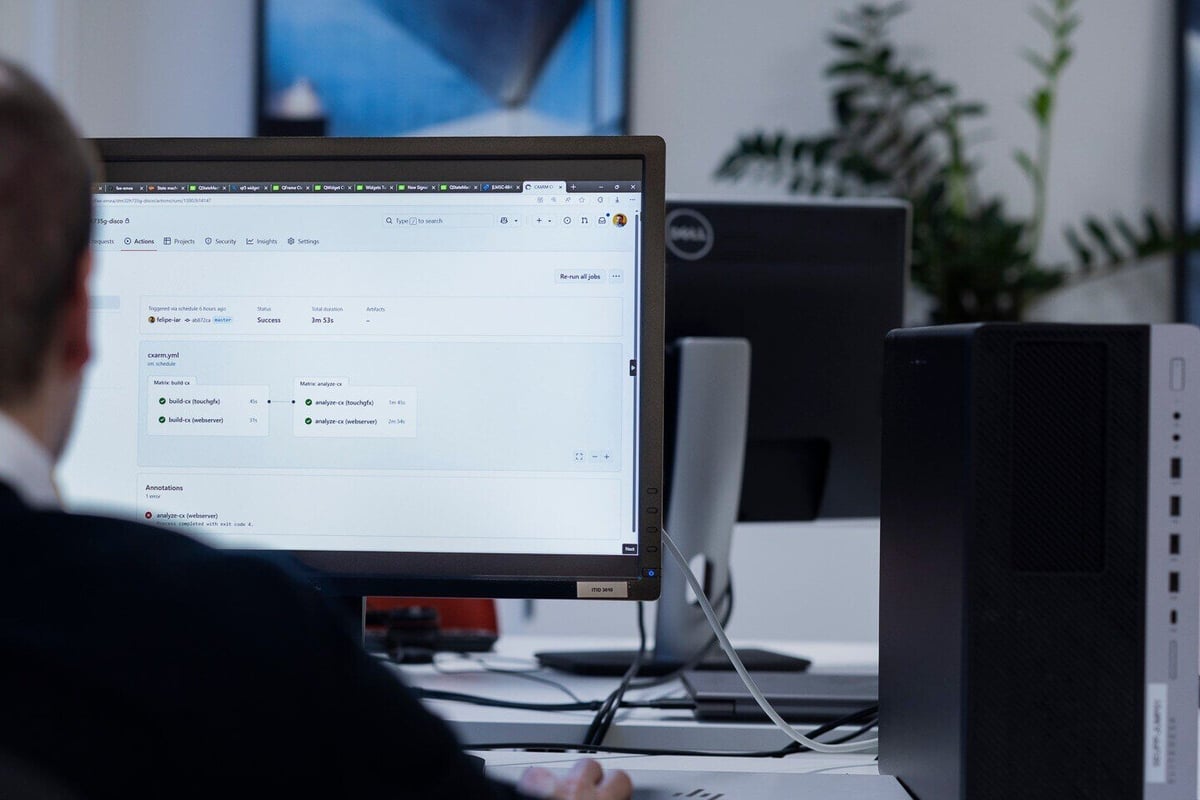
Embedded development
Learn more about embedded development and get the most out of our products in our articles, videos och recent webinars.
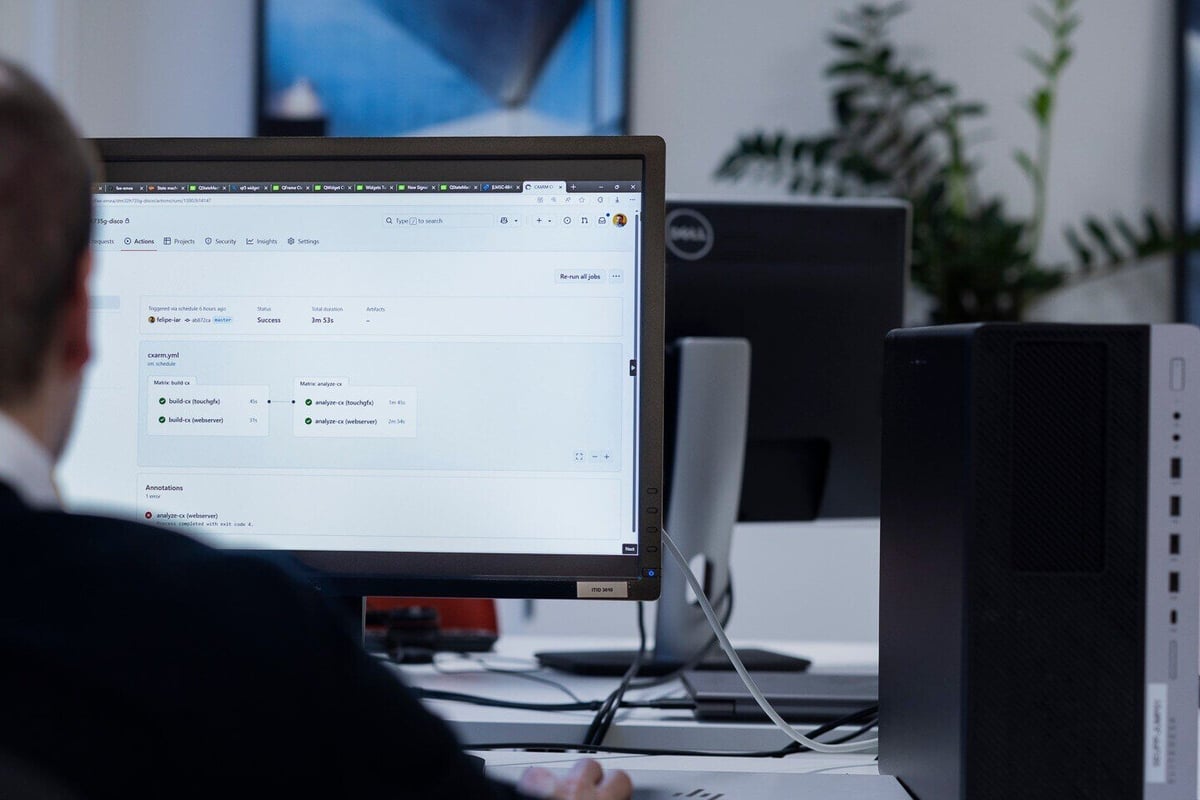
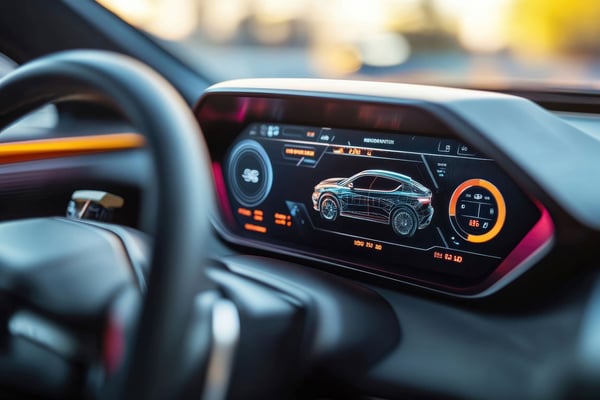
Why validating your own toolchain for functional safety is costly and time-consuming
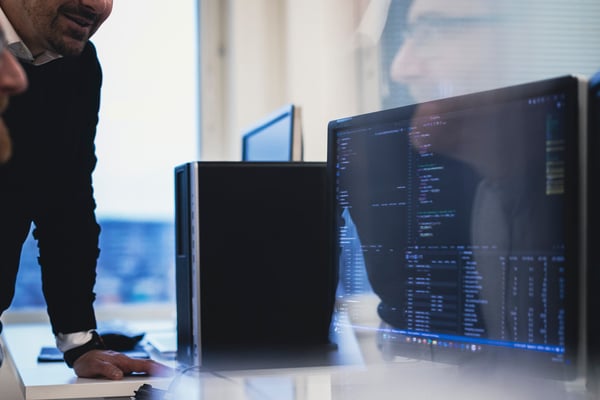
Basics of embedded development
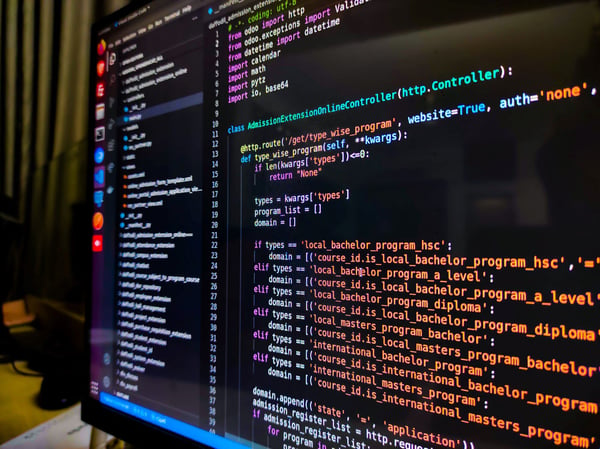
Code and data size for an RTOS-based design
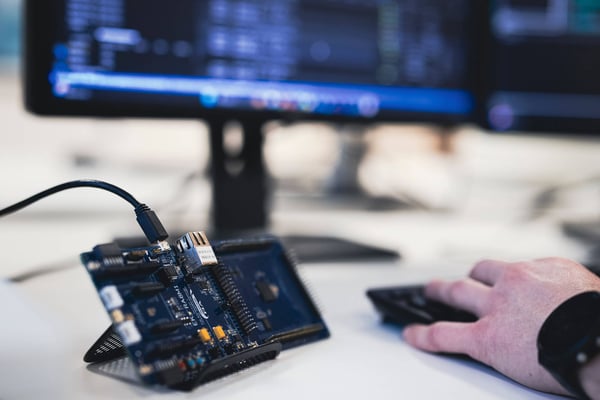
Finding bugs in Arm Cortex-M3 and -M4 applications
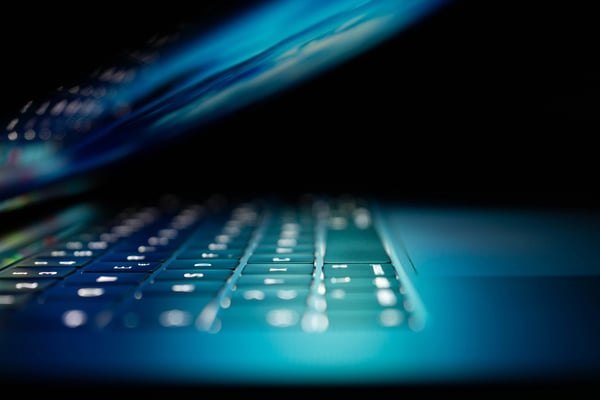
The straight way to secure device software applications
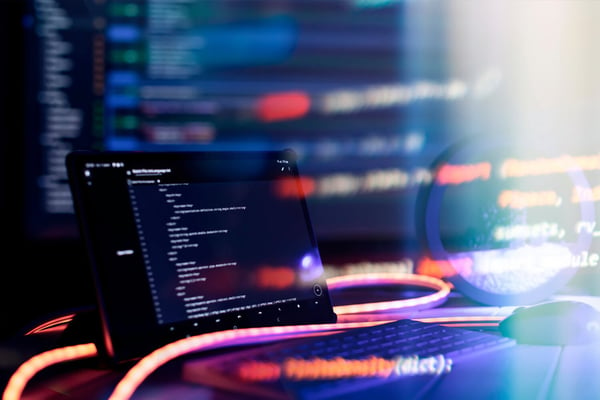
To C or not to C: deeper code insights through static analysis
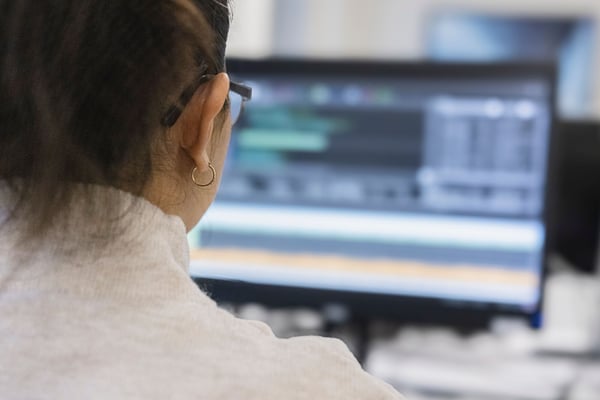
Move fast and break things? Not so fast in embedded
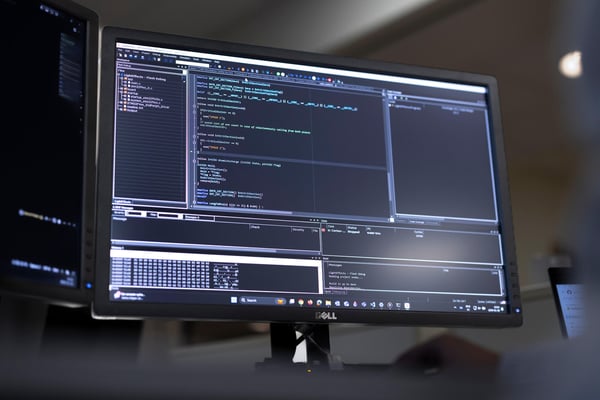
How to stay focused on your code
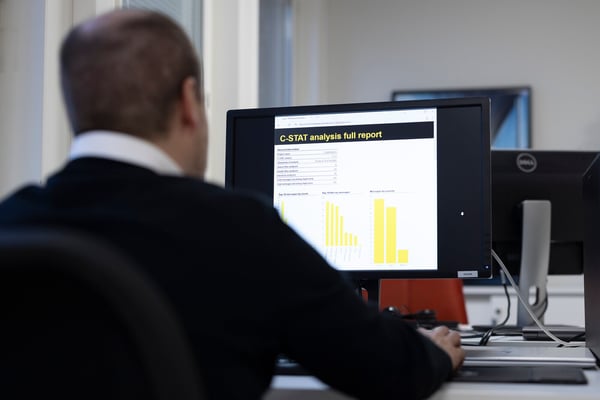